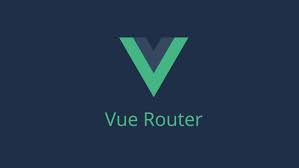
Building Single Page Applications with Vue Router
Key Features:
Nested Routes: Define routes within routes.
Dynamic Routing: Render components based on dynamic segments in the URL.
History Mode: Uses the History API to create clean URLs without hashes.
Setting Up Vue Router:
Installation:
1
npm install vue-router
Defining Routes:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
import Vue from 'vue'; import VueRouter from 'vue-router'; import Home from './components/Home.vue'; import About from './components/About.vue'; Vue.use(VueRouter); const routes = [ { path: '/', component: Home }, { path: '/about', component: About }, ]; const router = new VueRouter({ routes, mode: 'history', }); new Vue({ router, render: h => h(App), }).$mount('#app');
Using Router Links:
1 2 3 4 5 6 7 8 9
<template> <div id="app"> <nav> <router-link to="/">Home</router-link> <router-link to="/about">About</router-link> </nav> <router-view></router-view> </div> </template>