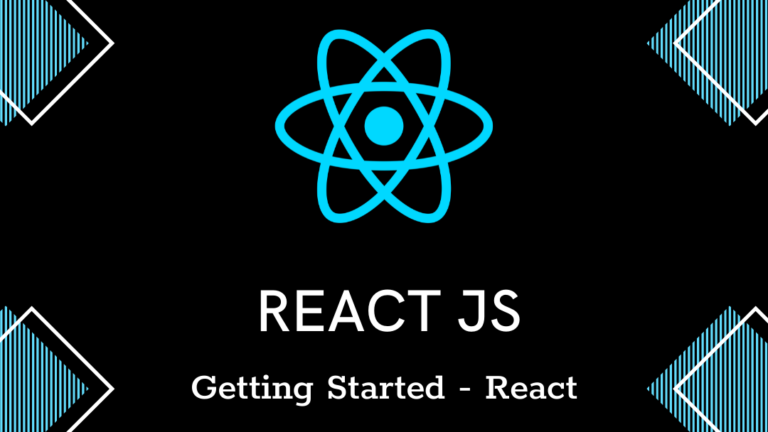
Getting Started with React: A Beginner's Guide
React is a JavaScript library for building user interfaces, developed and maintained by Facebook.
Key Concepts:
Components: Building blocks of a React application, can be class-based or functional.
JSX: JavaScript XML, a syntax extension that allows writing HTML in React.
State and Props: State is local to the component, while props are used to pass data between components.
Getting Started:
Installation:
1 2 3
npx create-react-app my-app cd my-app npm start
Creating a Component:
1 2 3 4 5 6 7
import React from 'react'; function Welcome(props) { return <h1>Hello, {props.name}</h1>; } export default Welcome;
Using the Component:
1 2 3 4 5
import React from 'react'; import ReactDOM from 'react-dom'; import Welcome from './Welcome'; ReactDOM.render(<Welcome name="World" />,document.getElementById('root'));